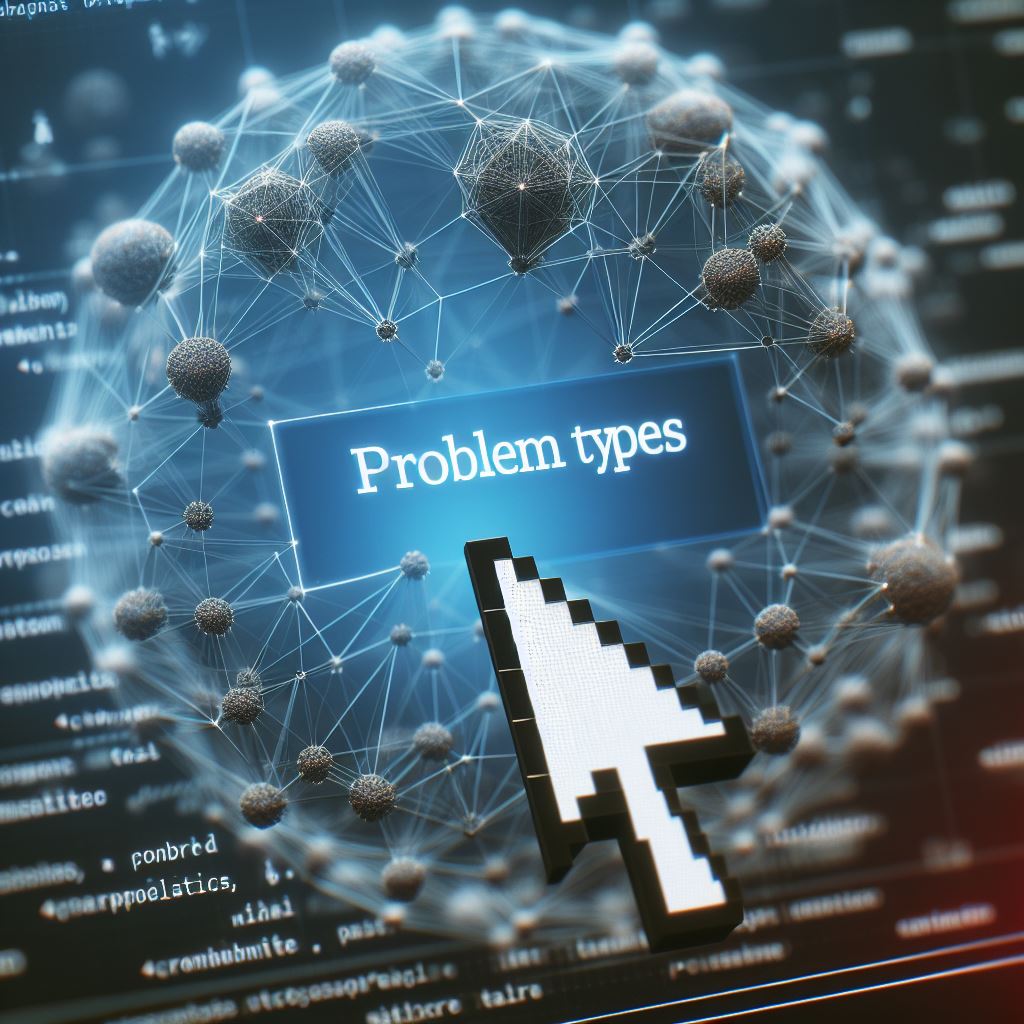
Problem Types &
Solving Approaches
In computing, while the range of problems is vast and diverse, they can generally be classified into several key categories to facilitate research efforts. By organizing problems into these categories, researchers can apply existing algorithms more effectively.
Some common problem types include:
Sorting: Sorting involves arranging a given set of items into a specific order, provided the nature of the items permits such an arrangement. For instance, sorting a set of numbers in ascending or descending order, or arranging character strings (such as names) alphabetically.
Searching: Searching involves finding a particular item or element within a given dataset. This could include locating a specific value in a list of numbers or finding a particular record in a database.
Graph problems: Graph problems revolve around analyzing and manipulating data represented as graphs. This includes tasks such as finding the shortest path between two nodes, determining whether a graph is connected, or identifying cycles within a graph.
Combinatorial problems: Combinatorial problems involve counting or selecting objects subject to certain constraints. Examples include calculating the number of possible arrangements of a set of items or determining the number of combinations that satisfy specific criteria.
Geometric problems: Geometric problems deal with properties and relationships of shapes and spatial structures. This encompasses tasks such as calculating areas, volumes, or distances, as well as determining intersections or overlaps between geometric entities.
Numerical problems: Numerical problems involve operations and manipulations on numbers. This can range from basic arithmetic computations to more complex numerical analysis tasks, such as solving equations or optimizing functions.
To evaluate algorithms in these categories, standard input sets are often defined as benchmarking sets. These sets enable researchers to analyze algorithm performance across various problem instances and dataset sizes, facilitating comparisons and optimizations.
Sorting Algorithms
Sorting is a fundamental process in computer science, involving the arrangement of a given set of items into a specific order, provided the nature of the items allows for such ordering. This can include sorting a set of numbers in ascending or descending order, or arranging character strings (e.g., names) alphabetically.
Researchers have developed numerous sorting algorithms tailored to different types of items. Not all sorting algorithms perform optimally for all types of item lists. Some excel in terms of resource usage, while others prioritize computational speed. The efficiency of a sorting algorithm also hinges on the input type; some algorithms perform well on randomly ordered inputs, while others are more efficient for nearly sorted lists. Additionally, certain algorithms are optimized for sorting data residing in memory, while others are better suited for sorting large files stored on secondary disks.
As of now, the most efficient sorting algorithms typically require nlogn comparisons to sort a list of n items.
A sorting algorithm is considered stable if it preserves the relative positions of equal items in the input list. In other words, if there are two equal items at positions i and j in the input list (where i < j), then in the sorted list, these items should retain their original order, with the item at position i appearing before the item at position j. Stable sorting algorithms ensure that equal items do not swap positions or interchange with each other during the sorting process.
the memory usage of a sorting algorithm is an important consideration, particularly when dealing with large datasets. While for small sets of items, the extra memory used during the swapping process might not be noticeable, it becomes significant for larger datasets. An algorithm is classified as in-place if it does not require a considerable amount of extra memory space beyond what is already allocated for the input data.
Two desirable characteristics for any sorting algorithm are stability and in-place operation.
In-place sorting algorithms manipulate the input data directly without needing to allocate additional memory for temporary storage during the sorting process. This characteristic is advantageous, especially when memory resources are limited or when dealing with exceptionally large datasets where minimizing memory usage is crucial for efficiency and performance.
By optimizing memory utilization, in-place sorting algorithms offer advantages in terms of reducing overall memory footprint and potentially enhancing processing speed, particularly in situations where memory resources are scarce or expensive. These algorithms play a significant role in various applications, ranging from sorting arrays in embedded systems with limited memory to sorting large datasets in distributed computing environments where minimizing memory overhead is essential for scalability and performance.
Searching Algorithms
Searching involves locating an element, known as a search key, within a set of items, which may contain duplicates. It's a critical operation performed on datasets and databases. The field of algorithm analysis often focuses on improving search algorithms, recognizing that no single algorithm is optimal for all scenarios. Factors such as speed, memory usage, and adaptability to different data types influence the design of search algorithms. Additionally, the nature of the data, whether static or dynamic, requires tailored approaches to searching, considering operations like addition or deletion of items.
String Processing
The proliferation of textual data, fueled by social media and blogging platforms, has spurred research interest in string-handling algorithms. This growth is also attributed to the commercial value of text data, particularly in predicting user interests for e-commerce purposes. Major search engines, including Google, heavily rely on string processing. Within this domain, string matching poses a significant challenge, reflecting the complexity of analyzing and manipulating textual data.
Sting matching is one of the string processing problems.
Graph Problems:
Researchers often find it advantageous to transform computational problems into graph problems due to the efficiency of graph-based solutions. Many computational challenges can be effectively addressed through graphs. For instance, tasks such as visiting all nodes in a graph (broadcasting in networks) or routing in networks (finding optimal paths like the shortest or minimum delay paths) can be efficiently solved using graph algorithms. Conversely, some graph problems pose computational challenges. The Traveling Salesman Problem (TSP), for instance, involves finding the shortest path that visits each of n cities exactly once. Another example is the graph-coloring problem, which aims to assign colors to vertices such that adjacent vertices have different colors, using the fewest number of colors possible. In the context of TSP, cities can be represented as vertices in the graph, highlighting the interconnectedness of these problems. Combinatorial problems encompass a multitude of potential solutions, often presenting a challenge due to the vast number of permutations and combinations. These problems span various domains, including scheduling, routing, and optimization. Examples such as the Traveling Salesman Problem, Independent Set Problem, and Subset Sum Problem illustrate the complexity inherent in combinatorial challenges, both in theoretical and practical contexts. Geometric algorithms find wide-ranging applications in fields such as computer graphics, robotics, and tomography. These algorithms tackle diverse geometric challenges, including the construction of shapes such as triangles, circles, and other geometric objects using basic tools like ruler and compass. Numerical computing encompasses a range of challenges including solving simultaneous linear equations (linear algebra), differential equations, definite integration, and statistical analysis. While many numerical problems are solvable, they often face a significant challenge: the accumulation of errors across multiple iterations. This accumulation occurs due to rounding off approximated results at each iteration, potentially leading to inaccuracies in the final solution.. These methodologies, often referred to as blind algorithms, involve systematically generating and evaluating every conceivable solution. They are characterized by their exponential or factorial time complexity.
The Divide and Conquer strategy is a fundamental algorithmic technique wherein a problem is recursively partitioned into smaller subproblems until they become trivial to solve. It follows a top-down approach, progressively breaking down the initial instance into smaller sub-instances through intermediate steps. The Greedy approach is widely used for efficiently solving optimization problems, where the objective is either maximization or minimization of a given set of input values, subject to specific constraints. In Greedy algorithms, the best choice at each step is selected to optimize the given objective, following a "greedy" approach. Dynamic Programming is a bottom-up approach that involves solving all subproblems, storing these intermediate results, and then reusing them to tackle larger subproblems until the solution to the original problem is achieved. The key advantage of dynamic programming lies in reusing the results of subproblems, thereby avoiding redundant computations and significantly reducing processing time compared to naive or straightforward methods. The Branch and Bound algorithm is an effective method for solving discrete and combinatorial optimization problems. In this approach, a search tree is constructed where each node represents a potential solution or subset of the solution set. The algorithm explores branches of this tree, systematically considering candidate solutions.
Randomized algorithms utilize randomness during the computation process, selecting random numbers at various stages to facilitate solution finding. This inherent randomness distinguishes them from deterministic algorithms, providing them with unique problem-solving capabilities.
The backtracking algorithm operates akin to creating checkpoints while traversing potential solutions. It follows a strategy similar to depth-first search, systematically exploring all possible solutions. During this exploration, if a solution fails to meet the criteria, the algorithm backtracks to the previous checkpoint and explores alternative paths to reach a viable solution. If no more alternative paths are available, the search concludes unsuccessfully.
Combinatorial Problems:
Due to the exponential growth in potential solutions with increasing input size, combinatorial problems pose significant computational hurdles, especially for large datasets. The sheer volume of possible combinations makes handling these problems daunting.
What adds to the difficulty is the scarcity of known algorithms capable of efficiently solving combinatorial problems within a reasonable timeframe. Many computer scientists contend that solving such problems optimally may be inherently infeasible.
Despite these challenges, a few fortunate exceptions exist where efficient solutions have been devised. For instance, algorithms for finding the shortest path in a network represent such exceptions, showcasing rare instances where combinatorial problems yield to effective algorithmic approaches.
Geometric Problems:
Within the realm of computational geometry, several classic problems are well-known:
Closest Pair Problem: This problem involves finding the closest pair of points from a given set in the plane.
Convex Hull Problem: Here, the objective is to construct the smallest convex polygon that encompasses all the points within a given set.
These problems represent fundamental challenges in computational geometry and have implications across various domains where geometric analysis is crucial.
Numerical Problems:
Problem Solving Techniques
Brute Force and Exhaustive Search Approaches:
To illustrate, consider the task of finding the correct four-letter word using Brute Force and Exhaustive Search Algorithms. As the problem's size increases, the number of potential outcomes escalates exponentially, rendering it practically infeasible to exhaustively enumerate all possibilities.
Divide and Conquer Approach:
Algorithms employing the Divide and Conquer technique typically involve the following steps:
Divide the problem at the top level into a set of sub-problems at a lower level.
Solve each sub-problem individually using a recursive approach.
Merge the solutions of the sub-problems to form a complete solution to the original problem.
Several problems can be efficiently tackled using the Divide and Conquer approach. Some examples include:
Binary Search
Quick Sort
Merge Sort
Strassen's Matrix Multiplication
Closest Pair of Points
Greedy Technique:
At each step, the Greedy method chooses the locally optimal solution, which may or may not result in the overall optimal solution. Consequently, the solution obtained through Greedy approach may not always be optimal but tends to be very close to the optimal solution.
Despite not guaranteeing the optimal solution in all cases, Greedy algorithms are often straightforward to design for optimization problems. Some examples of problems effectively solved using the Greedy approach include:
Kruskal's Minimum Spanning Tree
Prim's Minimal Spanning Tree
Dijkstra's Shortest Path
Knapsack Problem
Dynamic Programming:
The working principle of dynamic programming shares similarities with the divide and conquer approach. Both strategies break down a problem into several subproblems that can be recursively solved. However, dynamic programming overcomes the drawback of repetitive function calls inherent in divide and conquer by maintaining a table to store results. This dynamic decision-making process, whether to call a function or retrieve values from the table, justifies the term "dynamic" programming.
Dynamic programming outperforms divide and conquer by eliminating the redundancy of function calls with identical results. Examples of problems effectively solved using dynamic programming include the 0-1 Knapsack Problem and Subset-sum Problem.
Branch and Bound:
The Branch and Bound algorithm is an effective method for solving discrete and combinatorial optimization problems. In this approach, a search tree is constructed where each node represents a potential solution or subset of the solution set. The algorithm explores branches of this tree, systematically considering candidate solutions.
At each step, a candidate solution at a node is evaluated, and if it shows promise, it is further explored. However, if the candidate solution cannot produce a better outcome than the best one found so far, it is discarded. This pruning process helps in efficiently navigating through the solution space, potentially reducing the time complexity of the algorithm.Randomized Algorithms:
For instance, in Quick Sort, a random number can be chosen as the pivot during partitioning, helping to avoid worst-case scenarios and improving the overall efficiency of the sorting algorithm.
Similarly, when dealing with large numbers, a randomized algorithm may select a random number as a potential divisor for factoring, aiding in the decomposition of the number into its prime factors.Backtracking Algorithm:
In essence, backtracking involves iteratively attempting solutions, reverting to previous checkpoints when necessary, and exploring alternative paths until a satisfactory solution is found or all possibilities are exhausted. This method is particularly useful for problems with a large search space or combinatorial nature.